Problem description
Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target. You may assume that each input would have exactly one solution, and you may not use the same element twice. You can return the answer in any order.
Problem description explanation / breakdown
This tells us that we will have an array of numbers, for example, [2, 4, 3, 8, 6].
This also tells us that there is exactly one solution, meaning we will find only one pair of numbers that will add to the target.
This also tells us that we can return the answer in any order, meaning it doesn’t matter which of the two numbers that add to the target comes first or second in the array. For example, if we try to find a target “7,” and we have an array like this: [2, 4, 3, 8, 6], the answer is going to be “3” and “4” so we can return the answer like this [3, 4] or like this: [4, 3]. This clarification is important because it means there is always an answer, it doesn’t matter if there is more than one answer, but that there is an answer is what matters because if there was no answer we would have to account for that which will not be necessary in this problem.
Solution explanation
We will iterate through the array only once. Every single time we pass through each number, we will do one of two things, either check if the other number necessary to add up to the target already was passed, or if the other number has not been passed, we will save the current one.
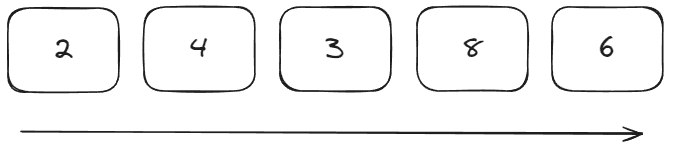
For example, let’s say our problem to solve is the following:
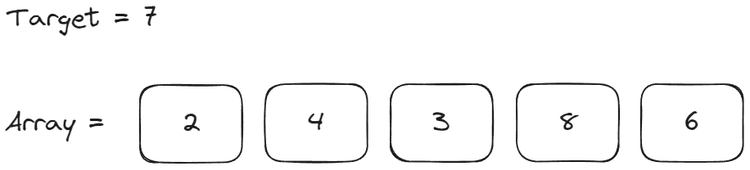
We will start with number 2, and check if the number 5 already exists in a container we are using to store the numbers we’ve gone through. We know it is 5 the one we are looking for because 7 - 2 = 5.
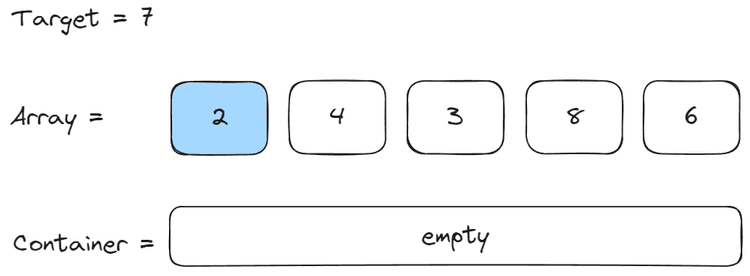
It is not present, so we store the number 2 and go to the next one.
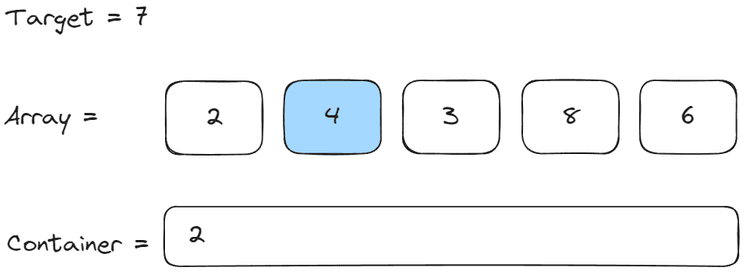
Now, we want to find a 3 since 7 - 4 = 3. There is no 3 in our container, so it means we haven’t gone through a 3. We save the number 4 in the container and go to the next number.
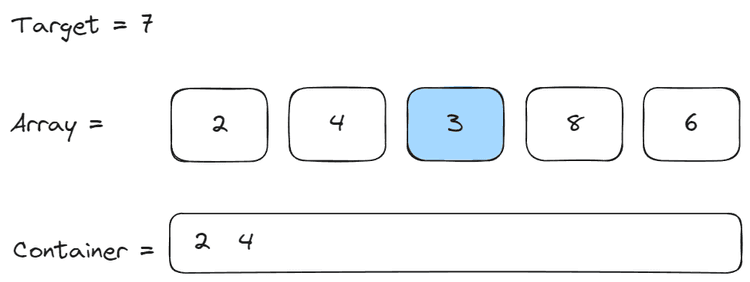
Now, we are looking for a 4 because 7 - 3 = 4. And now we do have it in our container, so it means we’ve found the answer. We were looking for two numbers that added to 7 and we found them, it’s 3 and 4. So that would be our answer.
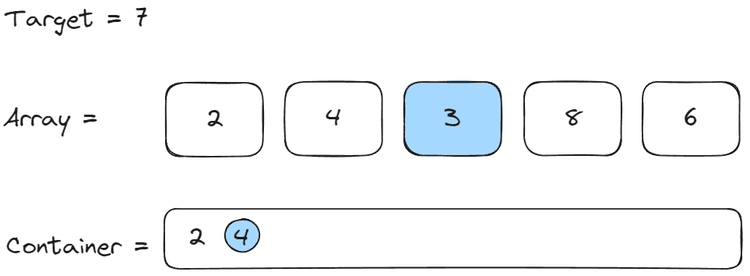
Pseudocode
Function twoSum(nums, target):
Let numIndicesMap be an empty hash map to store the indices of numbers.
For i from 0 to length of nums - 1:
Let complement be target - nums[i].
If complement exists in numIndicesMap:
Return [numIndicesMap[complement], i] # Indices of the two numbers found.
Else:
Add nums[i] and its index i to numIndicesMap.
# Example usage:
- nums = [2, 7, 11, 15]
- target = 9
- result = twoSum(nums, target)
- Print result # Output should be [2, 7] since nums[0] + nums[1] equals the target.
Code in C++
Complexity explanation
Space: O(n)
We are storing the elements and, in the worst-case scenario, the answer would be in the last two elements of the arrow, which means, we will have to store all the previous ones, which is the whole array.
Time: O(n)
We are iterating the array and, in the worst-case scenario, the answer would be in the last two elements, which means we would have to go through all the numbers until we reach the last two.